In this example we connect a L3G4200D three-axis gyroscope module to a Wemos Mini – you can of course use any ESP8266 based board but this is what I tested out.
First lets look at some information about the sensor from the manufacturer
The L3G4200D is a low-power three-axis gyroscope providing three different user selectable full scales (±250/±500/±2000 dps).It includes a sensing element and an IC interface able to provide the detected angular rate to the external world through a digital interface (I2C/SPI).
The sensing element is manufactured using specialized micromachining processes, while the IC interface is realized using a CMOS technology that allows designing a dedicated circuit which is trimmed to better match the sensing element characteristics.
Features
- Three selectable full scales (±250/500/2000 dps)
- I2C/SPI digital output interface
- 16 bit rate value data output
- Two digital output lines (interrupt and dataready)
- Integrated low and high pass filters with user selectable bandwidth
- Embedded self-test
- Wide supply voltage, 2.4 V to 3.6 V
- Low voltage compatible IOs, 1.8 V
- Embedded power-down and sleep mode
- High shock survivability
- Extended operating temperature range (-40 °C to +85 °C)
This was my sensor of choice that I used
Parts List
Here are the parts I used
Name | Links | |
Wemos Mini | ||
L3G4200D | ||
Connecting cables |
Schematic/Connection
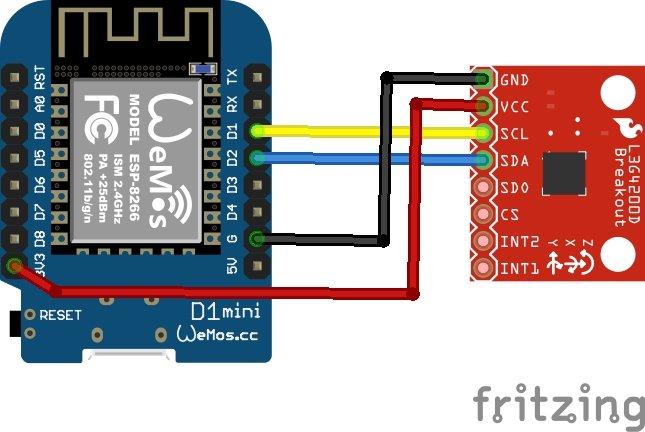
Code Example
This example uses the library – https://github.com/jarzebski/Arduino-L3G4200D
This is one of the default examples when you install the library above, there are quite a few to browse
/* L3G4200D Triple Axis Gyroscope. Simple Example. GIT: https://github.com/jarzebski/Arduino-L3G4200D Web: http://www.jarzebski.pl (c) 2014 by Korneliusz Jarzebski */ #include <Wire.h> #include <L3G4200D.h> L3G4200D gyroscope; void setup() { Serial.begin(9600); Serial.println("Initialize L3G4200D"); while(!gyroscope.begin(L3G4200D_SCALE_2000DPS, L3G4200D_DATARATE_400HZ_50)) { Serial.println("Could not find a valid L3G4200D sensor, check wiring!"); delay(500); } // Check selected scale Serial.print("Selected scale: "); switch(gyroscope.getScale()) { case L3G4200D_SCALE_250DPS: Serial.println("250 dps"); break; case L3G4200D_SCALE_500DPS: Serial.println("500 dps"); break; case L3G4200D_SCALE_2000DPS: Serial.println("2000 dps"); break; default: Serial.println("unknown"); break; } // Check Output Data Rate and Bandwidth Serial.print("Output Data Rate: "); switch(gyroscope.getOdrBw()) { case L3G4200D_DATARATE_800HZ_110: Serial.println("800HZ, Cut-off 110"); break; case L3G4200D_DATARATE_800HZ_50: Serial.println("800HZ, Cut-off 50"); break; case L3G4200D_DATARATE_800HZ_35: Serial.println("800HZ, Cut-off 35"); break; case L3G4200D_DATARATE_800HZ_30: Serial.println("800HZ, Cut-off 30"); break; case L3G4200D_DATARATE_400HZ_110: Serial.println("400HZ, Cut-off 110"); break; case L3G4200D_DATARATE_400HZ_50: Serial.println("400HZ, Cut-off 50"); break; case L3G4200D_DATARATE_400HZ_25: Serial.println("400HZ, Cut-off 25"); break; case L3G4200D_DATARATE_400HZ_20: Serial.println("400HZ, Cut-off 20"); break; case L3G4200D_DATARATE_200HZ_70: Serial.println("200HZ, Cut-off 70"); break; case L3G4200D_DATARATE_200HZ_50: Serial.println("200HZ, Cut-off 50"); break; case L3G4200D_DATARATE_200HZ_25: Serial.println("200HZ, Cut-off 25"); break; case L3G4200D_DATARATE_200HZ_12_5: Serial.println("200HZ, Cut-off 12.5"); break; case L3G4200D_DATARATE_100HZ_25: Serial.println("100HZ, Cut-off 25"); break; case L3G4200D_DATARATE_100HZ_12_5: Serial.println("100HZ, Cut-off 12.5"); break; default: Serial.println("unknown"); break; } // Calibrate gyroscope. The calibration must be at rest. // If you don't want calibrate, comment this line. gyroscope.calibrate(); // Set threshold sensivty. Default 3. // If you don't want use threshold, comment this line or set 0. gyroscope.setThreshold(3); } void loop() { // Read normalized values Vector raw = gyroscope.readRaw(); // Read normalized values in deg/sec Vector norm = gyroscope.readNormalize(); // Output raw Serial.print(" Xraw = "); Serial.print(raw.XAxis); Serial.print(" Yraw = "); Serial.print(raw.XAxis); Serial.print(" Zraw = "); Serial.print(raw.YAxis); // Output normalized Serial.print(" Xnorm = "); Serial.print(norm.XAxis); Serial.print(" Ynorm = "); Serial.print(norm.YAxis); Serial.print(" ZNorm = "); Serial.print(norm.ZAxis); Serial.println(); }
Output
Here is what I saw in Serial monitor, l moved the module around to see different values
Xraw = 42.00 Yraw = 42.00 Zraw = 65514.00 Xnorm = 0.00 Ynorm = 0.00 ZNorm = 0.00
Xraw = 45.00 Yraw = 45.00 Zraw = 65518.00 Xnorm = 0.00 Ynorm = 0.00 ZNorm = 0.00
Xraw = 111.00 Yraw = 111.00 Zraw = 65201.00 Xnorm = 0.00 Ynorm = 0.00 ZNorm = 0.00
Xraw = 1927.00 Yraw = 1927.00 Zraw = 65474.00 Xnorm = 0.00 Ynorm = 0.00 ZNorm = 0.00
Xraw = 1051.00 Yraw = 1051.00 Zraw = 65236.00 Xnorm = 0.00 Ynorm = 0.00 ZNorm = 0.00
Xraw = 878.00 Yraw = 878.00 Zraw = 790.00 Xnorm = 0.00 Ynorm = 0.00 ZNorm = 0.00
Xraw = 63084.00 Yraw = 63084.00 Zraw = 954.00 Xnorm = 0.00 Ynorm = 0.00 ZNorm = 0.00
Xraw = 262.00 Yraw = 262.00 Zraw = 2630.00 Xnorm = 0.00 Ynorm = 0.00 ZNorm = 0.00
Xraw = 65220.00 Yraw = 65220.00 Zraw = 62634.00 Xnorm = 0.00 Ynorm = 0.00 ZNorm = 0.00
Xraw = 65155.00 Yraw = 65155.00 Zraw = 133.00 Xnorm = 0.00 Ynorm = 0.00 ZNorm = 0.00
Xraw = 65102.00 Yraw = 65102.00 Zraw = 505.00 Xnorm = 0.00 Ynorm = 0.00 ZNorm = 0.00
Links