In this article we look at a VCNL4040 Proximity Sensor and we will connect it to a Wemos Mini
First lets look at the sensor
The VCNL4040 integrates a proximity sensor (PS), ambientlight sensor (ALS), and a high power IRED into one small package. It incorporates photodiodes, amplifiers, and analog to digital converting circuits into a single chip by CMOS process.
The 16-bit high resolution ALS offers excellent sensing capabilities with sufficient selections to fulfill most applications whether dark or high transparencylens design.
High and low interrupt thresholds can be programmed for both ALS and PS, allowing the component to use a minimal amount of the microcontrollers resources.
The proximity sensor features an intelligent cancellation scheme, so that cross talk phenomenon is eliminated effectively. To accelerate the PS response time, smart persistence prevents the misjudgment of proximity sensing but also keeps a fast response time.
In active force mode, a single measurement can be requested, allowing another good approach for more design flexibility to fulfill different kinds of applications with more power saving.
The patented Filtron technology achieves ambient light spectral sensitivity closest to real human eye response and offers the best background light cancellation capability(including sunlight) without utilizing the microcontrollers’resources.
VCNL4040 provides an excellent temperature compensation capability for keeping output stable undervarious temperature configurations. ALS and PS functions are easily set via the simple command format of I2C interface protocol.
Operating voltage ranges from 2.5 V to 3.6 V.
Here is the sensor I purchased from the good folks at Adafruit, there are other similar types of sensors available from other companies
Parts List
Here are the parts I used
Name | Links | |
Wemos Mini | ||
VCNL4040 | ||
Connecting cables |
Schematic/Connection
I used the Adafruit VCNL4040 sensor and in this case used the Stemma connection on the sensor
For the STEMMA QT cables, it uses the Qwiic convention:
Black for GND
Red for V+
Blue for SDA
Yellow for SCL
So color coded for ease of use, this layout shows a connection to the module
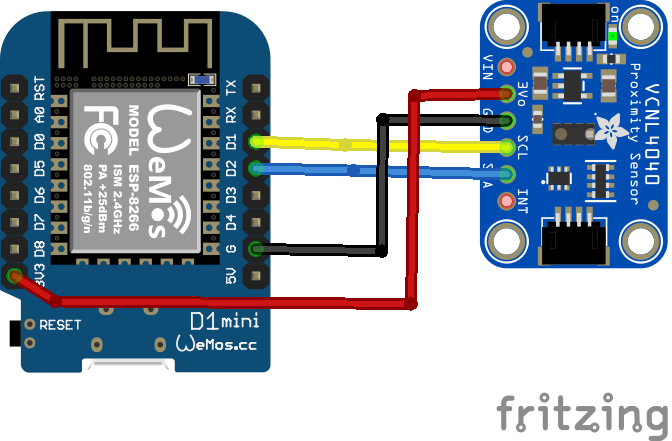
Code Example
This uses the library from Adafruit installed using the Library Manager in the Arduino IDE. search for VCNL4040 , and select the Adafruit VCNL4040 Library library
This is the default example
#include <Adafruit_VCNL4040.h> Adafruit_VCNL4040 vcnl4040 = Adafruit_VCNL4040(); void setup() { Serial.begin(115200); // Wait until serial port is opened while (!Serial) { delay(1); } Serial.println("Adafruit VCNL4040 Config demo"); if (!vcnl4040.begin()) { Serial.println("Couldn't find VCNL4040 chip"); while (1); } Serial.println("Found VCNL4040 chip"); //vcnl4040.setProximityLEDCurrent(VCNL4040_LED_CURRENT_200MA); Serial.print("Proximity LED current set to: "); switch(vcnl4040.getProximityLEDCurrent()) { case VCNL4040_LED_CURRENT_50MA: Serial.println("50 mA"); break; case VCNL4040_LED_CURRENT_75MA: Serial.println("75 mA"); break; case VCNL4040_LED_CURRENT_100MA: Serial.println("100 mA"); break; case VCNL4040_LED_CURRENT_120MA: Serial.println("120 mA"); break; case VCNL4040_LED_CURRENT_140MA: Serial.println("140 mA"); break; case VCNL4040_LED_CURRENT_160MA: Serial.println("160 mA"); break; case VCNL4040_LED_CURRENT_180MA: Serial.println("180 mA"); break; case VCNL4040_LED_CURRENT_200MA: Serial.println("200 mA"); break; } //vcnl4040.setProximityLEDDutyCycle(VCNL4040_LED_DUTY_1_40); Serial.print("Proximity LED duty cycle set to: "); switch(vcnl4040.getProximityLEDDutyCycle()) { case VCNL4040_LED_DUTY_1_40: Serial.println("1/40"); break; case VCNL4040_LED_DUTY_1_80: Serial.println("1/80"); break; case VCNL4040_LED_DUTY_1_160: Serial.println("1/160"); break; case VCNL4040_LED_DUTY_1_320: Serial.println("1/320"); break; } //vcnl4040.setAmbientIntegrationTime(VCNL4040_AMBIENT_INTEGRATION_TIME_80MS); Serial.print("Ambient light integration time set to: "); switch(vcnl4040.getAmbientIntegrationTime()) { case VCNL4040_AMBIENT_INTEGRATION_TIME_80MS: Serial.println("80 ms"); break; case VCNL4040_AMBIENT_INTEGRATION_TIME_160MS: Serial.println("160 ms"); break; case VCNL4040_AMBIENT_INTEGRATION_TIME_320MS: Serial.println("320 ms"); break; case VCNL4040_AMBIENT_INTEGRATION_TIME_640MS: Serial.println("640 ms"); break; } //vcnl4040.setProximityIntegrationTime(VCNL4040_PROXIMITY_INTEGRATION_TIME_8T); Serial.print("Proximity integration time set to: "); switch(vcnl4040.getProximityIntegrationTime()) { case VCNL4040_PROXIMITY_INTEGRATION_TIME_1T: Serial.println("1T"); break; case VCNL4040_PROXIMITY_INTEGRATION_TIME_1_5T: Serial.println("1.5T"); break; case VCNL4040_PROXIMITY_INTEGRATION_TIME_2T: Serial.println("2T"); break; case VCNL4040_PROXIMITY_INTEGRATION_TIME_2_5T: Serial.println("2.5T"); break; case VCNL4040_PROXIMITY_INTEGRATION_TIME_3T: Serial.println("3T"); break; case VCNL4040_PROXIMITY_INTEGRATION_TIME_3_5T: Serial.println("3.5T"); break; case VCNL4040_PROXIMITY_INTEGRATION_TIME_4T: Serial.println("4T"); break; case VCNL4040_PROXIMITY_INTEGRATION_TIME_8T: Serial.println("8T"); break; } //vcnl4040.setProximityHighResolution(false); Serial.print("Proximity measurement high resolution? "); Serial.println(vcnl4040.getProximityHighResolution() ? "True" : "False"); Serial.println(""); } void loop() { Serial.print("Proximity:"); Serial.println(vcnl4040.getProximity()); Serial.print("Ambient light:"); Serial.println(vcnl4040.getLux()); Serial.print("Raw white light:"); Serial.println(vcnl4040.getWhiteLight()); Serial.println(""); delay(500); }
Output
Open the serial monitor and you should see something like this.
Proximity:416
Ambient light:2
Raw white light:3
Proximity:1
Ambient light:22
Raw white light:26
Proximity:2
Ambient light:22
Raw white light:26
Links
https://www.vishay.com/docs/84274/vcnl4040.pdf
100+ ESP8266 Projects: Guides and Tutorials
https://lededitpro.com/ESP8266-projects