In this article we look at a SHT40 Temperature & Humidity Sensor and we will connect it to a Wemos Mini
First lets look at the sensor
This is the next generation of SHT sensor, in the past we have had examples for sensors such as the SHt31 and now we move on to the 4th generation of these
First lets look at some information about the sensor from the manufacturer
The SHT40 builds on a completely new and optimized CMOSens® chip that offers reduced power consumption and improved accuracy specifications. With the extended supply voltage range from 1.08 V to 3.6 V, it’s the perfect fit for mobile and battery-driven applications.
Size | 1.5 x 1.5 x 0.5 mm3 |
Output | I²C |
Supply voltage range | 1.08 to 3.6 V |
Energy consumption | 0.4µA (at meas. rate 1 Hz) |
RH operating range | 0 – 100% RH |
T operating range | -40 to +125°C (-40 to +257°F) |
RH response time | 6 sec (tau63%) |
Here is the sensor I purchased from the good folks at Adafruit
Unlike earlier SHT sensors, this sensor has a true I2C interface for easy interfacing with only two wires (plus power and ground!).
Thanks to the voltage regulator and level shifting circuitry that Adafruit have included on the breakout it is also 3V or 5V compliant, so you can plug it into the 5v of your Arduino without worrying about blowing the thing up – which is always handy.
Parts List
Here are the parts I used
Name | Links | |
Wemos Mini | ||
SHT40 | ||
Connecting cables |
Schematic/Connection
I used the Adafruit SHT40 sensor and in this case used the Stemma connection on the sensor
For the STEMMA QT cables, it uses the Qwiic convention:
Black for GND
Red for V+
Blue for SDA
Yellow for SCL
So color coded for ease of use, this layout shows a connection to the module
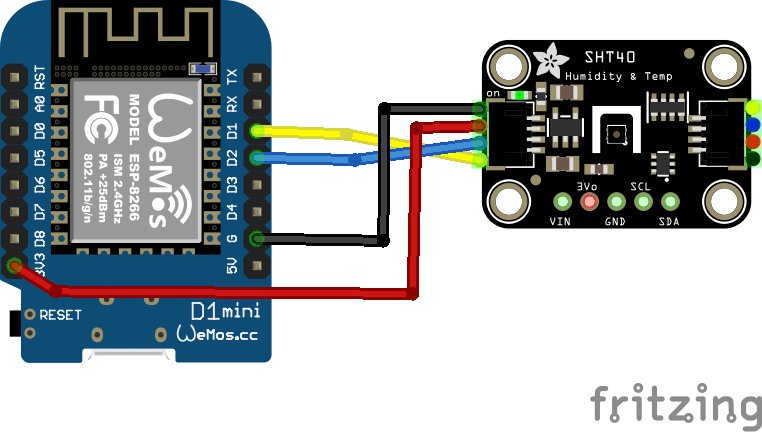
Code Example
This uses the library from Adafruit installed using the Library Manager in the Arduino IDE. search for SHT4x, and select the Adafruit SHT4x library and also the Adafruit BusIO library
This is the default example
/*************************************************** This is an example for the SHT4x Humidity & Temp Sensor Designed specifically to work with the SHT4x sensor from Adafruit ----> https://www.adafruit.com/products/4885 These sensors use I2C to communicate, 2 pins are required to interface ****************************************************/ #include "Adafruit_SHT4x.h" Adafruit_SHT4x sht4 = Adafruit_SHT4x(); void setup() { Serial.begin(115200); while (!Serial) delay(10); // will pause Zero, Leonardo, etc until serial console opens Serial.println("Adafruit SHT4x test"); if (! sht4.begin()) { Serial.println("Couldn't find SHT4x"); while (1) delay(1); } Serial.println("Found SHT4x sensor"); Serial.print("Serial number 0x"); Serial.println(sht4.readSerial(), HEX); // You can have 3 different precisions, higher precision takes longer sht4.setPrecision(SHT4X_HIGH_PRECISION); switch (sht4.getPrecision()) { case SHT4X_HIGH_PRECISION: Serial.println("High precision"); break; case SHT4X_MED_PRECISION: Serial.println("Med precision"); break; case SHT4X_LOW_PRECISION: Serial.println("Low precision"); break; } // You can have 6 different heater settings // higher heat and longer times uses more power // and reads will take longer too! sht4.setHeater(SHT4X_NO_HEATER); switch (sht4.getHeater()) { case SHT4X_NO_HEATER: Serial.println("No heater"); break; case SHT4X_HIGH_HEATER_1S: Serial.println("High heat for 1 second"); break; case SHT4X_HIGH_HEATER_100MS: Serial.println("High heat for 0.1 second"); break; case SHT4X_MED_HEATER_1S: Serial.println("Medium heat for 1 second"); break; case SHT4X_MED_HEATER_100MS: Serial.println("Medium heat for 0.1 second"); break; case SHT4X_LOW_HEATER_1S: Serial.println("Low heat for 1 second"); break; case SHT4X_LOW_HEATER_100MS: Serial.println("Low heat for 0.1 second"); break; } } void loop() { sensors_event_t humidity, temp; uint32_t timestamp = millis(); sht4.getEvent(&humidity, &temp);// populate temp and humidity objects with fresh data timestamp = millis() - timestamp; Serial.print("Temperature: "); Serial.print(temp.temperature); Serial.println(" degrees C"); Serial.print("Humidity: "); Serial.print(humidity.relative_humidity); Serial.println("% rH"); Serial.print("Read duration (ms): "); Serial.println(timestamp); delay(1000); }
Output
Open the serial monitor and you should see something like this.
Temperature: 24.59 degrees C
Humidity: 52.18% rH
Read duration (ms): 11
Temperature: 26.30 degrees C
Humidity: 52.92% rH
Read duration (ms): 11
Temperature: 27.46 degrees C
Humidity: 53.09% rH
Read duration (ms): 12
Links
https://learn.adafruit.com/introducing-adafruit-stemma-qt/technical-specs
https://www.sensirion.com/en/download-center/humidity-sensors/humidity-sensor-sht4x/