The MAX30102 is an integrated pulse oximetry and heart-rate monitor biosensor module. It includes internal LEDs, photodetectors, optical elements, and low-noise electronics with ambient light rejection. The MAX30102 provides a complete system solution to ease the design-in process for mobile and wearable devices.
The MAX30102 operates on a single 1.8V power supply and a separate 5.0V power supply for the internal LEDs. Communication is through a standard I2C-compatible interface. The module can be shut down through software with zero standby current, allowing the power rails to remain powered at all times.
Key Features
Heart-Rate Monitor and Pulse Oximeter Biosensor in LED Reflective Solution
Tiny 5.6mm x 3.3mm x 1.55mm 14-Pin Optical Module
Integrated Cover Glass for Optimal, Robust Performance
Ultra-Low Power Operation for Mobile Devices
Programmable Sample Rate and LED Current for Power Savings
Low-Power Heart-Rate Monitor (< 1mW)
Ultra-Low Shutdown Current (0.7µA, typ)
Fast Data Output Capability
High Sample Rates
Robust Motion Artifact Resilience
High SNR
-40°C to +85°C Operating Temperature Range
Parts List
Here are the parts I used
Name | Links | |
Wemos Mini | ||
MAX30102 | ||
Connecting cables |
Schematics/Layout
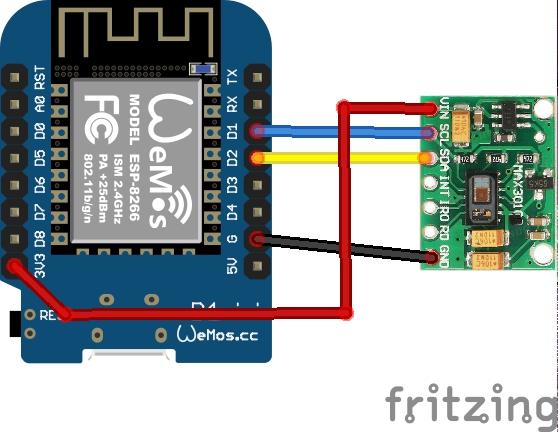
Code
Again we use a library and again its an sparkfun one – https://github.com/sparkfun/SparkFun_MAX3010x_Sensor_Library
#include <Wire.h> #include "MAX30105.h" #include "heartRate.h" MAX30105 particleSensor; const byte RATE_SIZE = 4; //Increase this for more averaging. 4 is good. byte rates[RATE_SIZE]; //Array of heart rates byte rateSpot = 0; long lastBeat = 0; //Time at which the last beat occurred float beatsPerMinute; int beatAvg; void setup() { Serial.begin(115200); Serial.println("Initializing..."); // Initialize sensor if (!particleSensor.begin(Wire, I2C_SPEED_FAST)) //Use default I2C port, 400kHz speed { Serial.println("MAX30105 was not found. Please check wiring/power. "); while (1); } Serial.println("Place your index finger on the sensor with steady pressure."); particleSensor.setup(); //Configure sensor with default settings particleSensor.setPulseAmplitudeRed(0x0A); //Turn Red LED to low to indicate sensor is running particleSensor.setPulseAmplitudeGreen(0); //Turn off Green LED } void loop() { long irValue = particleSensor.getIR(); if (checkForBeat(irValue) == true) { //We sensed a beat! long delta = millis() - lastBeat; lastBeat = millis(); beatsPerMinute = 60 / (delta / 1000.0); if (beatsPerMinute < 255 && beatsPerMinute > 20) { rates[rateSpot++] = (byte)beatsPerMinute; //Store this reading in the array rateSpot %= RATE_SIZE; //Wrap variable //Take average of readings beatAvg = 0; for (byte x = 0 ; x < RATE_SIZE ; x++) beatAvg += rates[x]; beatAvg /= RATE_SIZE; } } Serial.print("IR="); Serial.print(irValue); Serial.print(", BPM="); Serial.print(beatsPerMinute); Serial.print(", Avg BPM="); Serial.print(beatAvg); if (irValue < 50000) Serial.print(" No finger?"); Serial.println(); }
Output
Open the serial monitor – this is what I saw, it took a little bit of time for the readings to appear
IR=110798, BPM=93.46, Avg BPM=74
IR=110791, BPM=93.46, Avg BPM=74
IR=110879, BPM=93.46, Avg BPM=74
IR=110899, BPM=93.46, Avg BPM=74
IR=110969, BPM=93.46, Avg BPM=74
IR=111070, BPM=93.46, Avg BPM=74
IR=111021, BPM=93.46, Avg BPM=74
IR=110997, BPM=93.46, Avg BPM=74
IR=111116, BPM=93.46, Avg BPM=74
Links
https://datasheets.maximintegrated.com/en/ds/MAX30102.pdf
why i can found max30100 at fritzing? i already search from google but didnt found it
Same here – I just used an image of the module which can be added as an image and connect the wires. Not as easy as a part but still works OK
type MAX 30100 fzpz file download on google and then download the file and then click on the right side of the components part you will find an icon showing a downward arrow,Click on that and then click on the first option of import and then import the downloaded fzpz file to fritzing.
[…] MAX30102 pulse and heart-rate monitor sensor and ESP8266 […]
can you do it for spo2 also
Is it possible to use ESP-01 + MAX3010x ?
Is there any library that simulate I2C (SDA and SCL) on ESP-01?