This is another simple project using a Wemos MIni and a couple of shields. This time we will connect a DHT11 shield and an SD card shield and we will log some temperature data from the dht11 to an sd card. The data will be saved as a csv file, this means you can quite easily open in Microsoft Excel.
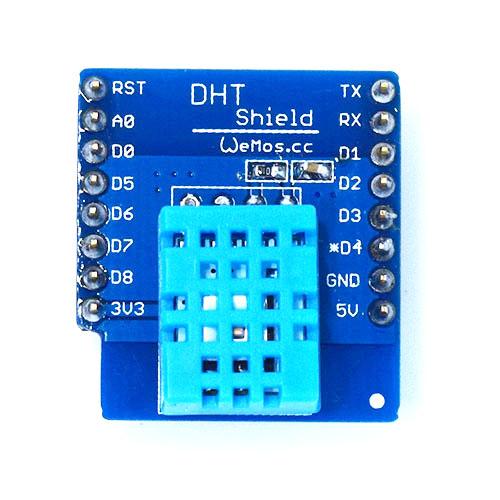
The 2 shields use the following I/O pins, the DHT uses D4 and the SD card uses the SPI pins D5, D6, D7 and D8
D1 mini | Shield |
D4 | DHT Pin |
D5 | SD CLK |
D6 | SD MISO |
D7 | SD MOSI |
D8 | SD CS |
Requirements
Wemos MIni
Micro SD Shield
DHT11 Shield
1 SD card ( I used an 8Gb one)
Code
[codesyntax lang=”cpp”]
#include "DHT.h" #include <SPI.h> #include <SD.h> #define DHTPIN D4 #define DHTTYPE DHT11 DHT dht(DHTPIN, DHTTYPE); const int chipSelect = D8; File myFile; void setup() { // Open serial communications and wait for port to open: Serial.begin(9600); while (!Serial) { ; // wait for serial port to connect. Needed for Leonardo only } Serial.print("Initializing SD card..."); if (!SD.begin(chipSelect)) { Serial.println("initialization failed!"); return; } Serial.println("initialization done."); dht.begin(); } void loop() { delay(2000); myFile = SD.open("dht11.csv", FILE_WRITE); // Reading temperature or humidity takes about 250 milliseconds! // Sensor readings may also be up to 2 seconds 'old' (its a very slow sensor) float h = dht.readHumidity(); float t = dht.readTemperature(); float f = dht.readTemperature(true); // Check if any reads failed and exit early (to try again). if (isnan(h) || isnan(t) || isnan(f)) { Serial.println("Failed to read from DHT sensor!"); return; } // if the file opened okay, write to it: if (myFile) { Serial.print("opened dht11.csv..."); myFile.print(h); myFile.print(","); myFile.print(t); myFile.print(","); myFile.println(f); // close the file: myFile.close(); Serial.println("closed dht11.csv."); } else { // if the file didn't open, print an error: Serial.println("error opening dht11.csv"); } }
[/codesyntax]
Testing
Open the csv file and you should see something like the following
34.00,25.00,77.00
34.00,25.00,77.00
34.00,25.00,77.00
34.00,25.00,77.00
34.00,25.00,77.00
34.00,25.00,77.00
34.00,25.00,77.00
33.00,25.00,77.00
33.00,25.00,77.00
33.00,25.00,77.00
33.00,25.00,77.00
Of course thats the data we wanted to log but you can add temperature in fahrenheit if you desired, perhaps some sort of time column
Links
Micro SD Shield for WeMos D1 mini TF
D1 Mini WeMos DHT11 Single Bus Digital Temperature Humidity Sensor Shield Module